Have you ever stared at your screen in frustration when your Mac or iOS app suddenly throws an error with Ukrainian text? You’re not alone.
The errordomain=nscocoaerrordomain&errormessage=не вдалося знайти вказану швидку команду.&errorcode=4 error isn’t just a mouthful—it’s a genuine headache for developers and users alike. This peculiar error strikes when your system can’t find a specific shortcut or file, bringing your workflow to a screeching halt.
Let’s crack this puzzle together. I’ll walk you through what this error means, why it happens, and give you practical solutions to get back on track. No fluff, just fixes.
What Does This NSCocoaErrorDomain Error Mean?
The errordomain=nscocoaerrordomain&errormessage=не вдалося знайти вказану швидку команду.&errorcode=4 error belongs to Apple’s Cocoa framework, which powers macOS and iOS applications. Breaking it down:
NSCocoaErrorDomain: Indicates the error comes from the Cocoa framework.
не вдалося знайти вказану швидку команду: Ukrainian for “could not find the specified shortcut.”
ErrorCode=4: In the Cocoa framework, error code 4 specifically relates to file not found issues.
When this error appears in your console or logs, it looks something like this:
Error Domain=NSCocoaErrorDomain Code=4 “не вдалося знайти вказану швидку команду.”
UserInfo={NSFilePath=/Users/username/Documents/missing_file.txt,
NSUnderlyingError=0x600003690f90 {Error Domain=NSPOSIXErrorDomain Code=2 “No such file or directory”}}
The most puzzling aspect? The Ukrainian error message appears regardless of your system language, often confusing developers who aren’t expecting non-English error messages.
Why Does This Error Occur? 5 Common Causes
The NSCocoaErrorDomain error with code 4 doesn’t just appear randomly. Here are the typical culprits behind this annoying interruption:
1. Missing Files or Invalid Paths
Your application is looking for a file that simply isn’t there anymore. This happens when:
swift// Problematic code – hard-coded path to a file that might not exist
let fileURL = URL(fileURLWithPath: “/Users/username/Documents/important_file.txt”)
do {
let contents = try String(contentsOf: fileURL, encoding: .utf8)
} catch {
print(“Error reading file: \(error)”)
}
Fix: Always check if a file exists before attempting to access it:
swiftlet fileURL = URL(fileURLWithPath: “/Users/username/Documents/important_file.txt”)
if FileManager.default.fileExists(atPath: fileURL.path) {
do {
let contents = try String(contentsOf: fileURL, encoding: .utf8)
} catch {
print(“Error reading file: \(error)”)
}
} else {
print(“File doesn’t exist at path: \(fileURL.path)”)
}
2. Permission Issues
Your app lacks the necessary permissions to access the file. This often happens with sandboxed apps or when accessing system-protected directories.
swift// Problematic code – attempting to write to a protected directory
let protectedURL = URL(fileURLWithPath: “/Library/System/file.txt”)
do {
try “Hello World”.write(to: protectedURL, atomically: true, encoding: .utf8)
} catch {
print(“Error writing file: \(error)”) // NSCocoaErrorDomain error 4 occurs here
}
Fix: Use proper directories that your app has access to:
swiftlet documentsDirectory = FileManager.default.urls(for: .documentDirectory, in: .userDomainMask).first!
let fileURL = documentsDirectory.appendingPathComponent(“file.txt”)
do {
try “Hello World”.write(to: fileURL, atomically: true, encoding: .utf8)
} catch {
print(“Error writing file: \(error)”)
}
3. Broken Aliases or Shortcuts
macOS aliases or symbolic links pointing to files that have been moved or deleted will trigger this error.
swift// Problematic code – following a symlink that might be broken
let symlinkURL = URL(fileURLWithPath: “/Users/username/Desktop/shortcut_link”)
do {
let destinationURL = try URL(resolvingAliasFileAt: symlinkURL)
// Try to access the file at destinationURL
} catch {
print(“Error resolving alias: \(error)”) // NSCocoaErrorDomain error occurs
}
Fix: Check if the symbolic link’s destination exists before following it:
swiftlet symlinkURL = URL(fileURLWithPath: “/Users/username/Desktop/shortcut_link”)
do {
let destinationURL = try URL(resolvingAliasFileAt: symlinkURL)
if FileManager.default.fileExists(atPath: destinationURL.path) {
// Safe to access the file
} else {
print(“Destination of alias does not exist”)
}
} catch {
print(“Error resolving alias: \(error)”)
}
4. File System Corruption
Sometimes, file system issues can cause this error even when the file should exist.
Fix: Run disk repair utilities:
Open Disk Utility
Select your drive
Click “First Aid”
Follow the prompts to repair any issues
5. Network or External Storage Problems
Files on disconnected network drives or external storage will trigger this error.
swift// Problematic code – hardcoded network path
let networkURL = URL(fileURLWithPath: “/Volumes/NetworkDrive/shared_file.txt”)
do {
let contents = try Data(contentsOf: networkURL)
} catch {
print(“Error reading network file: \(error)”) // NSCocoaErrorDomain error 4
}
Fix: Check connection status before accessing network resources:
swiftlet networkURL = URL(fileURLWithPath: “/Volumes/NetworkDrive/shared_file.txt”)
if FileManager.default.fileExists(atPath: networkURL.path) {
do {
let contents = try Data(contentsOf: networkURL)
} catch {
print(“Error reading network file: \(error)”)
}
} else {
print(“Network drive not connected or file doesn’t exist”)
Solutions Comparison: Prevention vs. Recovery
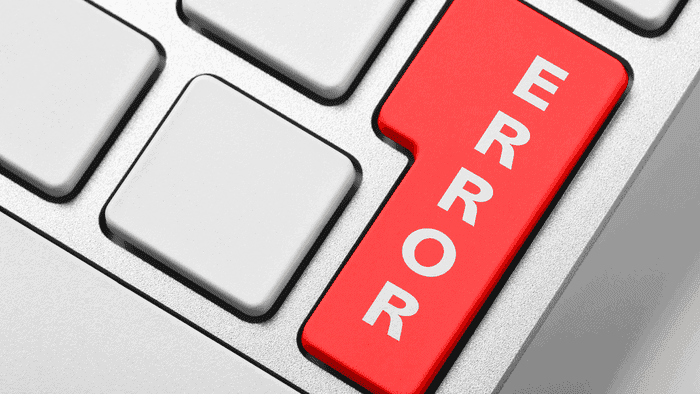
Prevention Techniques | Recovery Strategies |
Use FileManager.fileExists() before accessing files | Restore missing files from backups |
Implement proper error handling with try/catch | Repair permissions using Disk Utility or chmod commands |
Store file paths relatively, not absolutely | Rebuild broken aliases and shortcuts |
Request appropriate app sandbox entitlements | Reconnect network drives or external storage |
Implement fallback mechanisms for missing files | Use data recovery tools for corrupted files |
Step-by-Step Diagnosis
When you encounter this error, follow these systematic steps to pinpoint the exact cause:
Examine the complete error message
Look for the NSFilePath parameter in the error’s UserInfo dictionary. This tells you exactly which file the system couldn’t find.
swiftdo {
// Code that produces the error
} catch let error as NSError {
if error.domain == NSCocoaErrorDomain && error.code == 4 {
print(“File not found: \(error.userInfo[NSFilePathErrorKey] ?? “unknown path”)”)
}
}
Check file existence
Use FileManager to verify if the file exists at the expected path:
swiftlet path = error.userInfo[NSFilePathErrorKey] as? String ?? “”
if !FileManager.default.fileExists(atPath: path) {
print(“Confirmed: File does not exist at \(path)”)
}
Verify permissions
If the file exists but still produces the error, check permissions:
swiftlet path = error.userInfo[NSFilePathErrorKey] as? String ?? “”
if FileManager.default.isReadableFile(atPath: path) {
print(“File is readable”)
} else {
print(“Permission issue: File exists but is not readable”)
}
Check for alias or symbolic link issues
Determine if the path is an alias and if its target exists:
swiftlet url = URL(fileURLWithPath: path)
var isAlias: AnyObject?
do {
try (url as NSURL).getResourceValue(&isAlias, forKey: .isAliasFileKey)
if isAlias as? Bool == true {
print(“Path is an alias, checking destination…”)
do {
let destinationURL = try URL(resolvingAliasFileAt: url)
print(“Alias destination: \(destinationURL.path)”)
if !FileManager.default.fileExists(atPath: destinationURL.path) {
print(“Alias destination does not exist!”)
}
} catch {
print(“Failed to resolve alias: \(error)”)
}
}
} catch {
print(“Failed to check if path is alias: \(error)”)
}
Log error details for debugging
Create a comprehensive error log that captures all relevant details:
swiftfunc logFileError(_ error: Error, path: String) {
let nsError = error as NSError
let errorLog = “””
—- FILE ACCESS ERROR LOG —-
Error Domain: \(nsError.domain)
Error Code: \(nsError.code)
Description: \(nsError.localizedDescription)
File Path: \(path)
File Exists: \(FileManager.default.fileExists(atPath: path))
Is Readable: \(FileManager.default.isReadableFile(atPath: path))
Is Writable: \(FileManager.default.isWritableFile(atPath: path))
User Info: \(nsError.userInfo)
—————————–
“””
print(errorLog)
// You could also write this to a log file
}
Implementing a Robust File Access System
To prevent the NSCocoaErrorDomain error code 4 from haunting your app, implement this robust file access system:
swiftimport Foundation
// A comprehensive file handling class that prevents NSCocoaErrorDomain errors
class SafeFileManager {
enum FileError: Error {
case fileNotFound
case permissionDenied
case directoryCreationFailed
case aliasBroken
case networkDriveUnavailable
case unknown(Error)
}
static let shared = SafeFileManager()
private let fileManager = FileManager.default
// Safely read data from a file with comprehensive error handling
func readData(from path: String) -> Result<Data, FileError> {
1. Check if file exists
guard fileManager.fileExists(atPath: path) else {
return .failure(.fileNotFound)
}
2. Check permissions
guard fileManager.isReadableFile(atPath: path) else {
return .failure(.permissionDenied)
}
3. Handle aliases
let url = URL(fileURLWithPath: path)
var isAlias: AnyObject?
do {
try (url as NSURL).getResourceValue(&isAlias, forKey: .isAliasFileKey)
if isAlias as? Bool == true {
do {
let destinationURL = try URL(resolvingAliasFileAt: url)
// Recursively check the destination
return readData(from: destinationURL.path)
} catch {
return .failure(.aliasBroken)
}
}
} catch {
// Not an alias, continue
}
4. Handle network paths
if path.starts(with: “/Volumes/”) {
let components = path.components(separatedBy: “/”)
if components.count > 2 {
let volumePath = “/Volumes/\(components[2])”
var isVolumeMounted = false
do {
let volumes = try fileManager.contentsOfDirectory(atPath: “/Volumes”)
isVolumeMounted = volumes.contains(components[2])
} catch {
return .failure(.unknown(error))
}
if !isVolumeMounted {
return .failure(.networkDriveUnavailable)
}
}
}
5. Finally attempt to read the file
do {
let data = try Data(contentsOf: URL(fileURLWithPath: path))
return .success(data)
} catch {
return .failure(.unknown(error))
}
}
Write data to a file with full error handling
func writeData(_ data: Data, to path: String) -> Result<Void, FileError> {
// Create parent directories if needed
let url = URL(fileURLWithPath: path)
do {
if let parentDir = url.deletingLastPathComponent().path as String? {
if !fileManager.fileExists(atPath: parentDir) {
try fileManager.createDirectory(atPath: parentDir, withIntermediateDirectories: true)
}
}
} catch {
return .failure(.directoryCreationFailed)
}
// Check write permission on parent directory
let parentDir = url.deletingLastPathComponent().path
guard fileManager.isWritableFile(atPath: parentDir) else {
return .failure(.permissionDenied)
}
Try to write the file
do {
try data.write(to: url)
return .success(())
} catch {
return .failure(.unknown(error))
}
}
Get a safe path that’s guaranteed to be accessible
func getSafePath(for filename: String) -> String {
let documentsDirectory = fileManager.urls(for: .documentDirectory, in: .userDomainMask).first!
return documentsDirectory.appendingPathComponent(filename).path
}
Test file access without throwing exceptions
func canAccessFile(at path: String) -> Bool {
return fileManager.fileExists(atPath: path) && fileManager.isReadableFile(atPath: path)
}
}
Example Usage:
swift// Reading a file safely
let filePath = “/Users/username/Documents/important_file.txt”
switch SafeFileManager.shared.readData(from: filePath) {
case .success(let data):
print(“Successfully read \(data.count) bytes”)
case .failure(let error):
switch error {
case .fileNotFound:
print(“The file was not found at \(filePath)”)
// Implement recovery – perhaps look for the file in an alternate location
let alternativePath = SafeFileManager.shared.getSafePath(for: “important_file.txt”)
print(“Looking in alternative location: \(alternativePath)”)
case .permissionDenied:
print(“Permission denied accessing \(filePath)”)
// Show user how to grant permission
case .aliasBroken:
print(“The alias at \(filePath) is broken”)
// Offer to recreate the alias
case .networkDriveUnavailable:
print(“Network drive is unavailable”)
// Prompt user to reconnect network drive
case .unknown(let underlyingError):
print(“Unknown error: \(underlyingError)”)
default:
print(“Other error: \(error)”)
}
}
This implementation:
Prevents the NSCocoaErrorDomain error 4 by checking all possible failure points first
Provides specific error types for each failure scenario
Uses the Result type to handle success/failure cases without throwing exceptions
Includes helpful utilities like getting safe file paths
Has robust handling for aliases and network paths
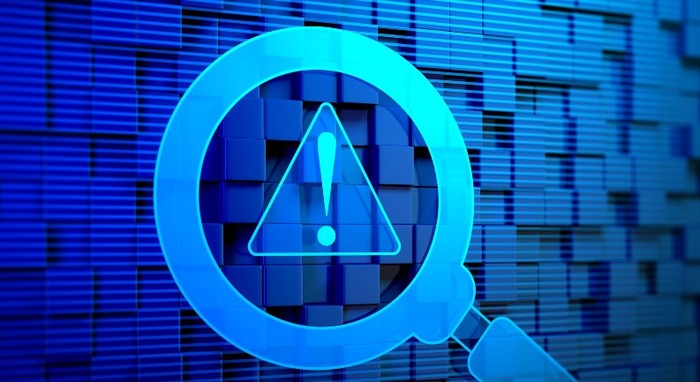
Conclusion
The errordomain=nscocoaerrordomain&errormessage=не вдалося знайти вказану швидку команду.&errorcode=4 error boils down to a file access issue. The most effective approach is preventative: implement robust file existence checking, proper error handling, and graceful fallbacks. When using file paths, prefer relative paths over absolute ones and verify resources exist before attempting to access them.
For developers, implementing the SafeFileManager class from this article will help you avoid this error while giving users a better experience when file access issues occur.